This code shows the RAM-Usage of the system;
using System;
using System.Collections;
using System.Threading;
using System.Diagnostics;
public class MyClass
{
public static void Main()
{
// Start Thread
Thread ramUsageThread = new Thread(new ThreadStart(GetRAMUsage));
ramUsageThread.IsBackground = true;
ramUsageThread.Start();
RL();
// End Thread
ramUsageThread.Abort();
WL("Thread stopped...\nPress any key to abort program.");
RL();
}
/* Thread-Method for RAM-Usage */
public static void GetRAMUsage()
{
PerformanceCounter pc = new PerformanceCounter("Memory", "Available Bytes");
do
{
// read next value
float byteCount = pc.NextValue();
Console.WriteLine("{0:0} Bytes available ({1:0} kB \t {2:0} MB)", byteCount, byteCount/1024, byteCount/(1024*1024));
// give a warning if available ram under 1 MB
if (byteCount < 1048576)
{
Console.WriteLine("WARNING: Available RAM under 1 MB!");
}
// stop thread a second
Thread.Sleep(1000);
} while (true);
}
#region Helper methods
private static void WL(object text, params object[] args)
{
Console.WriteLine(text.ToString(), args);
}
private static void RL()
{
Console.ReadLine();
}
private static void Break()
{
System.Diagnostics.Debugger.Break();
}
#endregion
}
Subscribe to:
Posts (Atom)
Nvidia's GauGan App
NVIDIA's GauGAN AI Machine Learning Tool creates photorealistic images from Simple Hand Doodling http://nvidia-research-mingyuliu.com/...
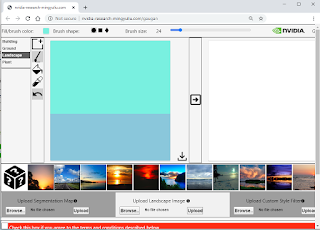
-
Code Snippet Index Page (from KTM Wiki) A Access DPI Access an External Database Active Page Index Add a Word Add an Alternative ...
-
CodeBlocks Arduino IDE is a customized distribution of the open-source Code::Blocks IDE enhanced for Arduino development. It provides mor...
-
Robocopy (Robust File Copy for Windows) is a very strong tool, which integrated in Windows since Vista. But the tool has lots of parameters ...